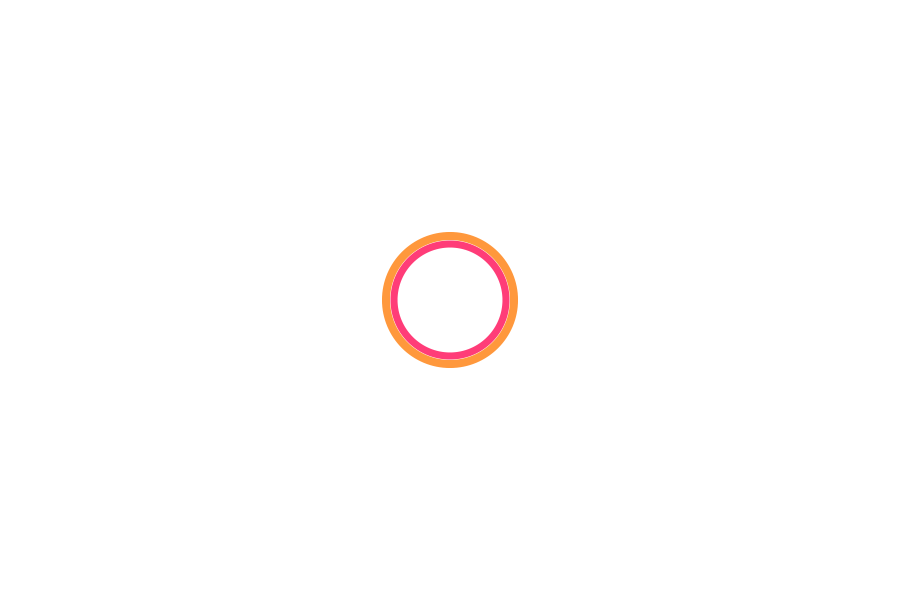
Flask Blueprint 組織程式碼的幾種方式
Blueprint 是一個用於標示架構和組織軟體專案的概念。在這裡,我們將探討三種不同的專案組織方式:單一應用程式、模組化應用程式,以及使用 Flask Blueprint 的應用程式。以下是這三種組織方式的程式範例:
按功能劃分藍圖
在這種組織方式中,我們根據應用程式的功能劃分藍圖。這個範例包含了一個簡單的 Flask 應用程式,使用了按功能劃分的 Blueprint 組織方式。應用程式有兩個功能模塊:身份驗證(auth)和文章(posts)。每個模塊都有自己的視圖和模板。在 app.py 中,我們註冊了兩個藍圖:auth_blueprint 和 posts_blueprint。
目錄結構:
1 2 3 4 5 6 7 8 9 10 11 12 13
| myproject/ ├── auth/ │ ├── __init__.py │ ├── views.py ├── posts/ │ ├── __init__.py │ ├── views.py ├── templates/ │ ├── auth/ │ │ ├── login.html │ ├── posts/ │ │ ├── list.html └── app.py
|
app.py:
1 2 3 4 5 6 7 8 9 10
| from flask import Flask from auth.views import auth_blueprint from posts.views import posts_blueprint
app = Flask(__name__) app.register_blueprint(auth_blueprint) app.register_blueprint(posts_blueprint)
if __name__ == '__main__': app.run()
|
auth/init.py:
auth/views.py:
1 2 3 4 5 6 7
| from flask import Blueprint, render_template
auth_blueprint = Blueprint('auth', __name__, template_folder='templates/auth')
@auth_blueprint.route('/login') def login(): return render_template('auth/login.html')
|
templates/auth/login.html:
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Login</title> </head> <body> <h1>Login Page</h1> </body> </html>
|
posts/init.py:
posts/views.py:
1 2 3 4 5 6 7
| from flask import Blueprint, render_template
posts_blueprint = Blueprint('posts', __name__, template_folder='templates/posts')
@posts_blueprint.route('/posts') def list_posts(): return render_template('posts/list.html')
|
templates/posts/list.html:
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Posts</title> </head> <body> <h1>Posts List</h1> </body> </html>
|
按角色劃分藍圖
在這種組織方式中,我們根據不同的使用者角色(如管理員、使用者…等)劃分藍圖。這樣可以讓應用程式的不同角色有各自的功能界面和邏輯。以下是按角色劃分 Blueprint 的簡單範例:
目錄結構:
1 2 3 4 5 6 7 8 9 10 11 12 13
| myproject/ ├── admin/ │ ├── __init__.py │ ├── views.py ├── user/ │ ├── __init__.py │ ├── views.py ├── templates/ │ ├── admin/ │ │ ├── dashboard.html │ ├── user/ │ │ ├── profile.html └── app.py
|
app.py:
1 2 3 4 5 6 7 8 9 10
| from flask import Flask from admin.views import admin_blueprint from user.views import user_blueprint
app = Flask(__name__) app.register_blueprint(admin_blueprint, url_prefix='/admin') app.register_blueprint(user_blueprint, url_prefix='/user')
if __name__ == '__main__': app.run()
|
admin/init.py:
admin/views.py:
1 2 3 4 5 6 7
| from flask import Blueprint, render_template
admin_blueprint = Blueprint('admin', __name__, template_folder='templates/admin')
@admin_blueprint.route('/dashboard') def dashboard(): return render_template('admin/dashboard.html')
|
templates/admin/dashboard.html:
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Admin Dashboard</title> </head> <body> <h1>Admin Dashboard</h1> </body> </html>
|
user/init.py:
user/views.py:
1 2 3 4 5 6 7
| from flask import Blueprint, render_template
user_blueprint = Blueprint('user', __name__, template_folder='templates/user')
@user_blueprint.route('/profile') def profile(): return render_template('user/profile.html')
|
templates/user/profile.html:
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>User Profile</title> </head> <body> <h1>User Profile</h1> </body> </html>
|
第一個範例按照角色劃分,有 admin 和 user 兩個角色,每個角色有對應的視圖和模板。
這些範例分別展示了按角色劃分和按資源劃分的兩種 Flask Blueprint 組織方式。
按資源類型劃分藍圖:
在這種組織方式中,我們根據應用程式中的資源類型(如文章、評論等)劃分藍圖。這樣可以讓程式碼更具模組化,便於維護和擴展。在 app.py 中,我們註冊了相應的藍圖。
目錄結構:
1 2 3 4 5 6 7 8 9 10 11 12 13
| myproject/ ├── posts/ │ ├── __init__.py │ ├── views.py ├── comments/ │ ├── __init__.py │ ├── views.py ├── templates/ │ ├── posts/ │ │ ├── list.html │ ├── comments/ │ │ ├── list.html └── app.py
|
app.py:
1 2 3 4 5 6 7 8 9 10
| from flask import Flask from posts.views import posts_blueprint from comments.views import comments_blueprint
app = Flask(__name__) app.register_blueprint(posts_blueprint, url_prefix='/posts') app.register_blueprint(comments_blueprint, url_prefix='/comments')
if __name__ == '__main__': app.run()
|
posts/init.py:
posts/views.py:
1 2 3 4 5 6 7
| from flask import Blueprint, render_template
posts_blueprint = Blueprint('posts', __name__, template_folder='templates/posts')
@posts_blueprint.route('/') def list_posts(): return render_template('posts/list.html')
|
templates/posts/list.html:
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Posts</title> </head> <body> <h1>Posts List</h1> </body> </html>
|
comments/init.py:
comments/views.py:
1 2 3 4 5 6 7
| from flask import Blueprint, render_template
comments_blueprint = Blueprint('comments', __name__, template_folder='templates/comments')
@comments_blueprint.route('/') def list_comments(): return render_template('comments/list.html')
|
templates/comments/list.html:
1 2 3 4 5 6 7 8 9 10
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Comments</title> </head> <body> <h1>Comments List</h1> </body> </html>
|
比較三種方案
下表對比了使用 Flask Blueprint 組織程式碼的三種方式的優缺點:
方式 |
優點 |
缺點 |
按功能劃分藍圖 |
功能模塊清晰 |
功能模塊過多時結構可能變得複雜 |
|
有利於專案的擴展性和可維護性 |
|
按角色劃分藍圖 |
便於實現角色之間的隔離和權限控制 |
角色之間存在相似功能時可能導致程式碼重複 |
|
有利於專案的擴展性和可維護性 |
|
按資源類型劃分藍圖 |
程式碼具有高度模組化 |
資源之間有相互依賴時可能需要更多的協調和溝通 |
|
便於維護和擴展 |
|
在選擇組織方式時,應該根據專案的需求和規模來決定。有時候,可以將這些組織方式結合使用,以便更好地滿足專案需求。
混合使用
綜合以上三種使用 Flask Blueprint 的程式碼組織方式,開發者可以根據專案需求靈活選擇或混合應用。在實際應用中,專案可能需要更細緻的組織結構,此時可以將多種組織方式結合起來,以便更好地滿足需求。
例如,我們可以根據應用程式的功能和資源類型同時劃分藍圖:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| myproject/ ├── auth/ │ ├── __init__.py │ ├── views.py ├── admin/ │ ├── posts/ │ │ ├── __init__.py │ │ ├── views.py │ ├── images/ │ │ ├── __init__.py │ │ ├── views.py ├── user/ │ ├── posts/ │ │ ├── __init__.py │ │ ├── views.py │ ├── comments/ │ │ ├── __init__.py │ │ ├── views.py ├── templates/ │ ├── auth/ │ │ ├── login.html │ │ ├── register.html │ ├── admin/ │ │ ├── posts/ │ │ │ ├── manage_posts.html │ │ ├── images/ │ │ │ ├── manage_images.html │ ├── user/ │ │ ├── posts/ │ │ │ ├── list.html │ │ │ ├── detail.html │ │ ├── comments/ │ │ │ ├── list.html │ │ │ ├── new_comment.html └── app.py
|
在這個範例中,我們同時根據功能(身份驗證、管理員、使用者)和資源類型(文章、圖片、評論)劃分藍圖。這樣可以使程式碼更具模組化,便於維護和擴展。同時,不同角色的功能和權限也得到了清晰的區分。